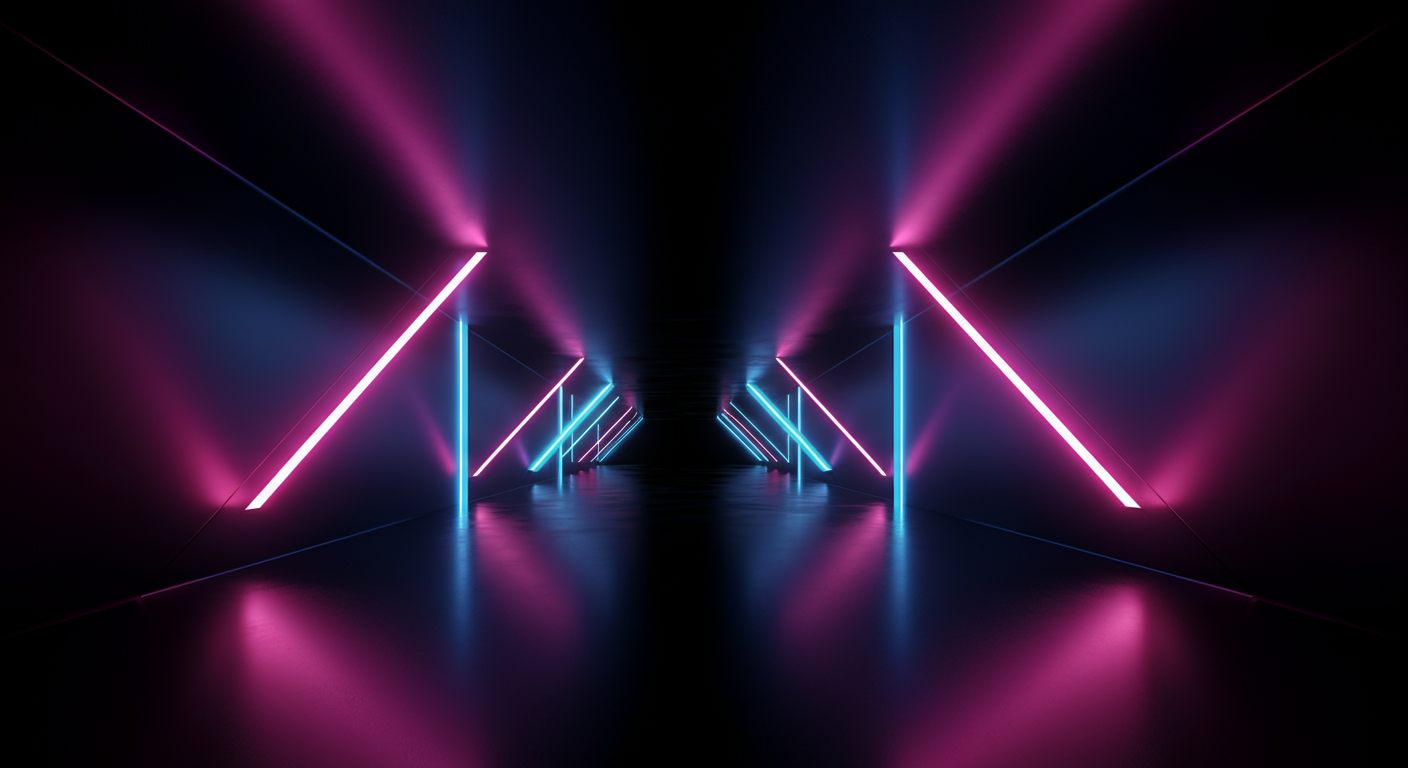
Step1 STEM
BONUS ANSWERS
If you want to check your Step1 BONUS challenge circuit and code, or you just need some help in completing the challenge, please look below!
const int redPin = 6; // Define the pin for the redLED const int greenPin = 7; // Define the pin for the green LED const int yellowPin = 8; // Define the pin for the yellow LED const int longDelayTime = 5000; // Delay time in milliseconds which is 5 seconds here const int shortDelayTime = 2000; // Delay time in milliseconds which is 2 seconds void setup() { pinMode(redPin, OUTPUT); // Set the red LED pin as an output pinMode(greenPin, OUTPUT); // Set the green LED pin as an output pinMode(yellowPin, OUTPUT); // Set the yellow LED pin as an output digitalWrite(redPin, LOW); // Start with the RED light off digitalWrite(greenPin, LOW); // Start with the GREEN light off digitalWrite(yellowPin, LOW); // Start with the YELLOW light off } void loop() { digitalWrite(redPin, HIGH); // Turn the red LED on delay(longDelayTime); // Wait for 5 seconds digitalWrite(redPin, LOW); // Turn the red LED off digitalWrite(greenPin, HIGH); // Turn the green LED on delay(longDelayTime); // Wait for 5 seconds digitalWrite(greenPin, LOW); // Turn the green LED off digitalWrite(yellowPin, HIGH); // Turn the yellow LED on delay(shortDelayTime); // Wait for 2 seconds digitalWrite(yellowPin, LOW); // Turn the yellow LED off // The loop will then start all over again starting with turning the RED LED on again }
Congratulations on making it this far, even if you needed some help in getting here! You have successfully completed the Step1 STEM course. At this point you should have a pretty solid understanding of some of the basics of writing code and interacting with an Arduino.
You may not realize it, but with all that you have learned in this program, you essentially have the basic skills to create the following products!
You have learned how to accept input from a user and then turn a servo motor back and forth. That is the basics to understanding how a remote control car works!
Further classes will explore how the remote control portion works.
You have learned how to setup, load and trigger MP3 files to play audio through a speaker. That is exactly how an MP3 audio player works!
You have learned how to write code to turn lights on and off in a special sequence. That is essentially how traffic lights work!